This program is a slightly more advanced version of a program found in the IDE Quick Start Manual (found here), you should start by going through that first as it will explain in more detail some of the things I will be doing here as well how to set up an apache web server from which to run the program. This time instead of displaying the current date and time we will be creating a very basic HTML page. The end result should hopefully look something like this
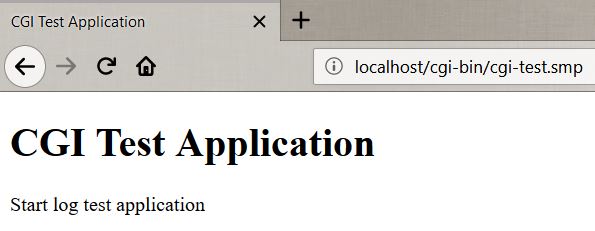
Setup
Before we can start doing anything programming we have to configure the project settings. We will first have to add theinclude
directory. The Project Settings Includes and libraries tab should then look like this:
At this point, we should set the target for our program so we will later be able to run the program in a web browser. It is good practice to not only include a target for executing the program but also one for debugging it. The standard target for our apache installation is: C:\Program Files (x86)\Ampps\www\cgi-bin\
this will be the same target for execution and debugging. The two shebang lines we need are as follows:

For execution: #!C:\SIMPOL\bin\smpcgi32.exe{d}{a}
For debugging: #!C:\SIMPOL\bin\sbngidecaller.exe{d}{a}
Base Program
Variable Declaration
For this basic program, we will require two variables one will be declared inside the function, the other will be found inside the function declaration
include "htmlheaders.sma"
function main(cgicall cgi)
string result;
cgi.output(sHTML_UTF8HEADER, 1);
end function result
The first thing to note here is the include statement, this adds the file htmlheaders.sma
into this program, this document contains several constants relevant to HTML headers (you can take a look at this file by double-clicking on it in the project view or by selecting it on the bottom set of tabs underneath our code. We are making use of this included file to declare the charset. This is done using the output function of our CGI variable:
cgi.output(sHTML_UTF8HEADER, 1);
The format for this function is explained in more detail in the language reference but for this purpose, you will only need to know that you need to parse HTML code into it, and in our case, we are also parsing a char size into the function.
Creating Web Page
At this point, we’re ready to write the main body of HTML code for our web page
cgi.output("<html>", 1);
cgi.output("<header>", 1);
cgi.output("<title>CGI Test Application</title>", 1);
cgi.output("</header>", 1);
cgi.output("<body><h1>CGI Test Application</h1>", 1);
It is not within the scope of this tutorial to explain the HTML code. Furthermore, the actual HTML used is not very important. The important thing to notice is again the use of cgi.output()
to write to the webpage
After we have done this compiling the program will allow us to access the webpage in your browser, assuming you have your Apache server running. At the moment it will only show you a website with a title and a renamed browser tab. To include a body we are going to be using a different method by making use of our result string instead of a function. This will allow us to write more complex code onto our page and use traditional logic to put change the information displayed. This can only happen when you return the string at the end of our function. However, anything more complex than that below is out of the scope of this basic program
result = "Start log test application"
One last point to make for this basic version is that whenever you execute this program there will be an error message, this is because we have not included a command line for our CGI. It is not necessary to include a command-line for this program
To launch the program you have to go to where you have stored the program= if you have downloaded the attached version this would be http://localhost/cgi-bin/cgi.smp.
The CGI/ISAPI/FastCGI Component (Old)
Code
include "htmlheaders.sma"
function main(cgicall cgi)
string result
cgi.output(sHTML_UTF8HEADER, 1);
cgi.output("<html>", 1);
cgi.output("<header>", 1);
cgi.output("<title>CGI Test Application</title>", 1);
cgi.output("</header>", 1);
cgi.output("<body><h1>CGI Test Application</h1>", 1);
result = "Start log test application"
end function result